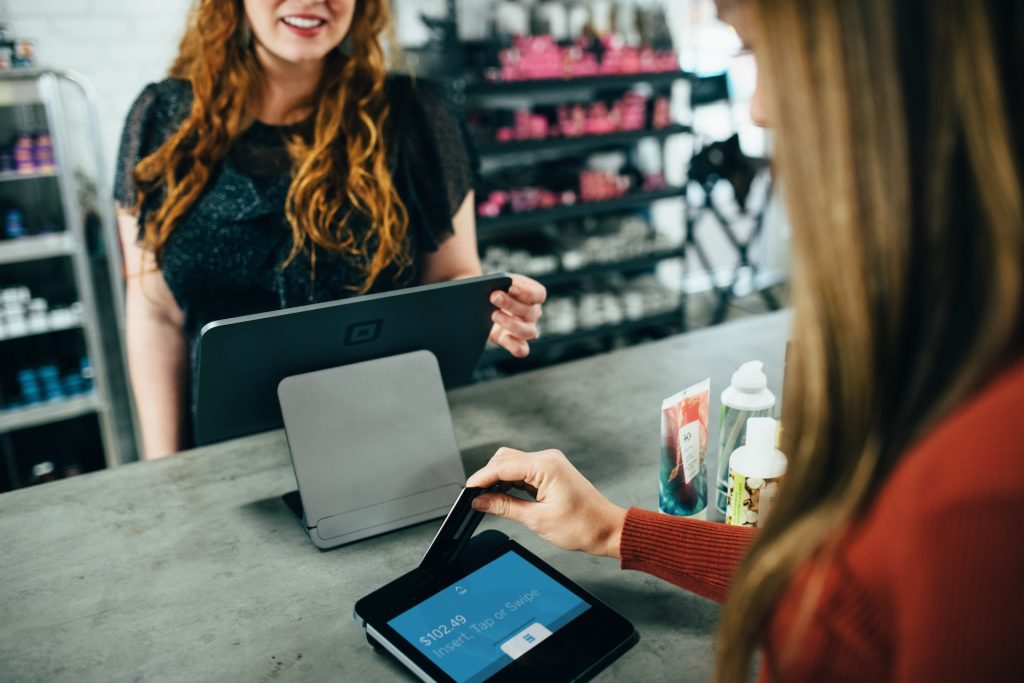
Why Escrow Payments are a Game-Changer in the World of E-Commerce
In the world of online transactions, security and trust are paramount. Implementing escrow payments adds an extra layer of protection for both buyers and sellers, ensuring that funds are safely held until predefined conditions are met. Stripe, a leading online payment platform, offers a robust infrastructure for integrating escrow payments seamlessly. In this comprehensive guide, we’ll walk you through the steps to implement escrow payments in Stripe, ensuring a secure and transparent online transaction process.
Step 1: Set Up Your Stripe Account for Escrow Payments
Before diving into the escrow implementation, ensure you have a fully functional Stripe account. Head over to the Stripe dashboard (https://dashboard.stripe.com) and sign in or create an account if you haven’t already. Once logged in, navigate to the “Developers” section and access your API keys.
Step 2: Integrate Stripe into Your Platform
Integrating Stripe into your website or application is the first crucial step. Utilize one of Stripe’s client libraries or plugins for popular frameworks to simplify the integration process. Follow the documentation provided by Stripe to ensure a seamless connection.
Step 3: Understand the Escrow Payments Workflow
To implement an escrow payments system, you must grasp the workflow. In a typical scenario, a buyer initiates a transaction, funds are held in escrow, and the seller fulfills the requirements. Only when the conditions are met is the payment released to the seller. Familiarize yourself with Stripe’s PaymentIntents API, which allows you to manage payments and handle escrow transactions.
Step 4: Implement PaymentIntents API
Before you start, make sure you have the Stripe PHP library installed. You can install it using Composer:
composer require stripe/stripe-php
Here’s a simple example to include and configure stripe php SDK into a project.
<?php
// Make sure to adjust the path based
// on your project structure
require 'vendor/autoload.php';
\Stripe\Stripe::setApiKey('YOUR_STRIPE_SECRET_KEY');
Now create a customer by using Stripe SDK for your Escrow Payments.
// Create a customer (buyer)
$customer = \Stripe\Customer::create([
'email' => 'buyer@example.com',
]);
Create a product effortlessly with the Stripe SDK, tailored for seamless integration into upcoming escrow transactions.
// Create a product
$product = \Stripe\Product::create([
'name' => 'Example Product',
]);
// Create a price for the product
$price = \Stripe\Price::create([
'product' => $product->id,
'unit_amount' => 1000, // amount in cents
'currency' => 'usd',
]);
Now create stripe payment intent.
// Create a payment intent
$paymentIntent = \Stripe\PaymentIntent::create([
'amount' => $price->unit_amount,
'currency' => $price->currency,
]);
// Display payment information to the buyer
echo "Product Price: " . number_format($price->unit_amount / 100, 2) . " " . $price->currency . PHP_EOL;
echo "You can pay using this PaymentIntent ID: " . $paymentIntent->id . PHP_EOL;
Step 5: Handle Escrow Payments Confirmation
Once the buyer initiates the payment, you’ll receive a client secret from the PaymentIntent creation. Use this secret to confirm the payment on the client side, triggering the payment authentication process. Keep in mind that the funds are still in escrow at this stage.
// Simulate payment completion (This would typically be done by the buyer)
$paymentIntent = \Stripe\PaymentIntent::retrieve($paymentIntent->id);
$paymentIntent->confirm();
Step 6: Fulfillment and Release of Funds
Now it’s time to transfer funds to the seller. Implement the necessary logic on your server to confirm when the seller has fulfilled the required conditions. When this happens, use the Transfer API to release them to the seller. This step completes the escrow process, ensuring a secure and transparent transaction.
// Transfer funds to the seller
$transfer = \Stripe\Transfer::create([
'amount' => $price->unit_amount,
'currency' => $price->currency,
'destination' => 'SELLER_STRIPE_ACCOUNT_ID',
]);
echo "Payment completed and funds transferred to the seller." . PHP_EOL;
Make sure to replace ‘YOUR_STRIPE_SECRET_KEY’ and ‘SELLER_STRIPE_ACCOUNT_ID’ with your actual Stripe secret key and the seller’s Stripe account ID.
Remember that this is a basic example, and a real-world escrow payments system would require more features, error handling, and security measures. It’s also important to comply with Stripe’s terms of service and any relevant regulations in your jurisdiction.
By following these steps, you can successfully implement an escrow system in Stripe, enhancing the security and trustworthiness of your online transactions. Remember to stay informed about any updates or changes to Stripe’s API, and regularly test your implementation to ensure a smooth and reliable escrow process for your users.